AP Computer Science A FRQ Answer 2024: Simulating a Bird Feeder (Question 1, Part A) – Step-by-Step Solution
- Sanjivani Singh
- Dec 1, 2024
- 4 min read
Updated: Dec 3, 2024
Looking for detailed AP Computer Science A FRQ answers?
In this post, we’ll guide you through a comprehensive, step-by-step solution to Question 1 (Part A) of the 2024 AP Computer Science A exam. By breaking down the problem into manageable steps, you’ll learn how to approach FRQs effectively. Once you understand this approach, you’ll be able to tackle any FRQ in your upcoming test with confidence. All the best!
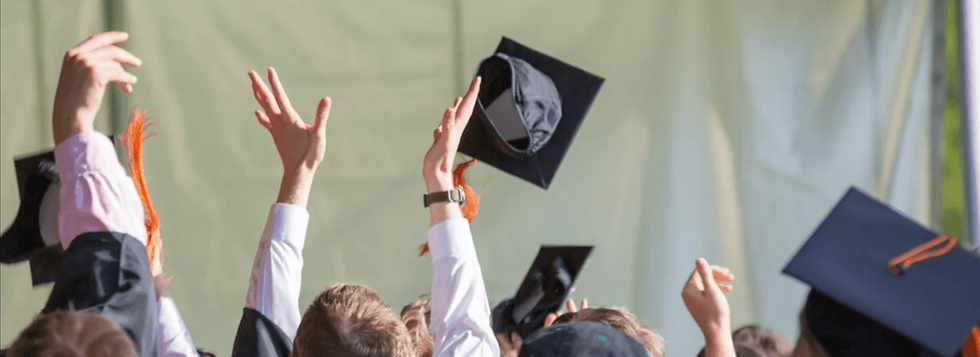
Introduction: Overview of the Problem
In this problem, we are asked to simulate a bird feeder with two possible scenarios: one where birds eat food from the feeder (95% of the time) and another where a bear comes and consumes all the food (5% of the time). We need to write a method in the Feeder class that handles these cases and updates the food in the feeder accordingly.
Problem Breakdown
The problem can be divided into two main conditions:
normal
abnormal.
Here’s how we’ll handle both:
Condition | Scenario | Chance | Food Consumed | Food left |
Normal Conditions | Birds consume food | 95% | Random between 10-50 grams per bird | Decreases by total food consumed |
Abnormal Conditions | A bear eats all the food in the feeder | 5% | All the food in the feeder | 0 grams |
Step-by-Step Solution Approach:
Step 1: Understand the Requirements
We need to simulate one day at the bird feeder, and depending on whether it’s a normal or abnormal day, we’ll adjust the amount of food in the feeder. The two scenarios are:
Normal conditions (95% of the time): Each bird eats a random amount of food between 10 and 50 grams.
Abnormal conditions (5% of the time): A bear eats all the food.
Step 2: Generate Random Scenarios (Normal vs. Abnormal)
We need to simulate whether it’s a normal day or an abnormal day. For that, we generate a random number between 0 and 99:
If the number is less than 95, it's a normal day (birds).
If the number is 95 or greater, it’s an abnormal day (bear).
Code for Random Scenario Generation:
// Random number between 0 and 99
int randomChance = (int)(Math.random() * 100);
Step 3: Handle Normal Day (Birds Eating)
For a normal day, each bird will eat a random amount of food between 10 and 50 grams. We then calculate the total food consumed by all the birds and adjust the feeder's food amount.
How much food each bird eats: A random value between 10 and 50 grams.
Total food consumed: Multiply the food each bird eats by the number of birds (numBirds).
Formula for Random Food Consumption:
// Formula for Random Food Consumption
// Random food per bird (between 10 and 50 grams)
int foodPerBird = 10 + (int)(Math.random() * 41);
// Total food eaten by all birds
int totalFoodEaten = numBirds * foodPerBird;
Step 4: Handle Edge Case (Food Runs Out)
If the total food consumed by the birds is greater than the currentFood in the feeder, the feeder is emptied (i.e., the food amount is set to 0).
// Code for Checking and Updating Food
if (totalFoodEaten > currentFood) {
// If more food is eaten than available, set food to 0
currentFood = 0;
} else {
// Otherwise, subtract the total food eaten from currentFood
currentFood -= totalFoodEaten;
}
Step 5: Handle Abnormal Day (Bear Eating)
On an abnormal day, the bear comes and eats all the food, so we simply set the currentFood to 0.
Code for Bear Eating:
currentFood = 0; // Bear eats all the food
Full Solution Code
Here’s the complete simulateOneDay method combining all the steps discussed:
public void simulateOneDay(int numBirds) {
// Step 1: Generate a random number to decide if it's a normal day or abnormal (bear).
int randomChance = (int)(Math.random() * 100); // Random number between 0 and 99
if (randomChance < 95) { // Normal day: 95% chance
// Step 2: Normal day: Birds eat the food
// Random food per bird (between 10 and 50)
int foodPerBird = 10 + (int)(Math.random() * 41);
// Total food eaten by all birds
int totalFoodEaten = numBirds * foodPerBird;
// Step 3: Check if the food consumed exceeds the food
available
if (totalFoodEaten > currentFood) {
// If more food is eaten than available, set food to 0
currentFood = 0;
} else {
// Subtract the total food eaten from current food
currentFood -= totalFoodEaten;
}
} else { // Abnormal day: 5% chance, bear eats all the food
currentFood = 0; // Bear eats all the food
}
}
Key Concepts Covered
Random Number Generation: Simulating real-world uncertainty (birds or bear).
Edge Cases: Handling situations where there’s not enough food for the birds.
Food Consumption Simulation: Both normal and abnormal conditions.
Explanation of the Code
Random Chance (Normal vs Abnormal Day):
We use Math.random() to generate a number between 0 and 99. If the number is less than 95, birds consume the food. If the number is 95 or greater, the bear eats all the food.
Normal Day (Birds Eating):
For birds, we randomly decide how much food each bird consumes (between 10 and 50 grams). We multiply this by the number of birds and update the feeder's food accordingly.
Abnormal Day (Bear Eating):
If it’s a bear, we immediately set the feeder's food to 0.
Additional Tips for Students
Practice with Edge Cases:
Consider scenarios where the number of birds is high and the food is low. What happens when birds try to consume more than what's available?
Efficiency:
Since the AP Computer Science A exam is conducted offline, so practice solving problems on paper. Take a few examples, write them out, and work step-by-step to see if your solution gives the correct results for different situations.
These steps are the breakdown for solving AP Computer Science A FRQ answers related to simulating a bird feeder.
Link to Official AP FRQ 2024
To access the official AP Computer Science A FRQ 2024 and other questions, refer to the official College Board resource:
Have Any Questions or Comments?
If you have any questions or comments about this solution or need further clarification, feel free to leave them in the comments section below! I'm happy to help and would love to hear your thoughts.
Comentarios